Custom Taxonomies are a great way to organise content and should be added to WordPress via a plugin so if a theme is swapped the taxonomies are maintained. Taxonomies are either hierarchal similar to ‘Categories’ or flat, similar to ‘Tags’.
You can assign custom taxonomies to regular posts as well as custom post types.
Create the plugin header and file in /wp-content/plugins either as a standalone .php file or structured inside a directory.
<?php /* Plugin Name: WP Beaches Taxonomies Plugin URI: http://wpbeaces.com/ Description: WP Beaches Custom Taxonomies Author: Neil Gee Version:1 Author URI:https://wpbeaches.com */
Once you have done this the plugin will appear in the Plugins list, it just needs to be activated, but we need to give it some functionality first.
Next up is to create the function and action to load the custom taxonomy
function themename_custom_taxonomies() { //code goes here } add_action( 'init', 'themename_custom_taxonomies' );
Here the function is declared themename_custom_taxonomies and the action init will execute it – now we just need to populate the function.
function themename_custom_taxonomies() { // Coaching Method $args = array( 'hierarchical' => true, 'labels' => $coach_method, 'show_ui' => true, 'query_var' => true, 'rewrite' => array( 'slug' => 'coach-method' ) ); register_taxonomy( 'coach-method', array( 'post' ), $args ); } add_action( 'init', 'themename_custom_taxonomies', 0 );
So here we are registering a new taxonomy ‘coach-method‘ and assigning it to all posts, this is defined in the first array with ‘post’, to assign the taxonomy to other custom post types you add them in that array separated by commas like so:
function themename_custom_taxonomies() { // Coaching Method $args = array( 'hierarchical' => true, 'labels' => $coach_method, 'show_ui' => true, 'query_var' => true, 'rewrite' => array( 'slug' => 'coach-method' ) ); register_taxonomy( 'coach-method', array( 'coach_mentor', 'post' ), $args ); } add_action( 'init', 'themename_custom_taxonomies', 0 );
Here the same taxonomy is assigned to posts as well as a CPT (custom post type) named ‘coach_mentor’. The $args array declares if the taxonomy is hierarchal, defines the label values (more in a minute on this), makes it visible, queryable and sets the url slug.
Labels
Labels set the names in the backend WP Admin interface, so it is best to use real names of what the taxonomy is rather than the generic defaults, the above array sets the value of labels as $coach_method, so the $coach_method labels need to be defined in another array within the same function.
function themename_custom_taxonomies() { // Coaching Method $coach_method = array( 'name' => _x( 'Coach Method', 'taxonomy general name' ), 'singular_name' => _x( 'Coach Method', 'taxonomy singular name' ), 'search_items' => __( 'Search in Coach Methods' ), 'all_items' => __( 'All Coach Methods' ), 'most_used_items' => null, 'parent_item' => null, 'parent_item_colon' => null, 'edit_item' => __( 'Edit Coach Method' ), 'update_item' => __( 'Update Coach Method' ), 'add_new_item' => __( 'Add new Coach Method' ), 'new_item_name' => __( 'New Coach Method' ), 'menu_name' => __( 'Coach Method' ), ); $args = array( 'hierarchical' => true, 'labels' => $coach_method, 'show_ui' => true, 'query_var' => true, 'rewrite' => array( 'slug' => 'coach-method' ) ); register_taxonomy( 'coach-method', array( 'coach_mentor', 'post' ), $args ); } add_action( 'init', 'themename_custom_taxonomies', 0 );
This will take care of all the labels. Now also add another taxonomy within the same function and set it as a flat structure similar to tags.
function themename_custom_taxonomies() { // Coaching Method $coach_method = array( 'name' => _x( 'Coach Method', 'taxonomy general name' ), 'singular_name' => _x( 'Coach Method', 'taxonomy singular name' ), 'search_items' => __( 'Search in Coach Methods' ), 'all_items' => __( 'All Coach Methods' ), 'most_used_items' => null, 'parent_item' => null, 'parent_item_colon' => null, 'edit_item' => __( 'Edit Coach Method' ), 'update_item' => __( 'Update Coach Method' ), 'add_new_item' => __( 'Add new Coach Method' ), 'new_item_name' => __( 'New Coach Method' ), 'menu_name' => __( 'Coach Method' ), ); $args = array( 'hierarchical' => true, 'labels' => $coach_method, 'show_ui' => true, 'query_var' => true, 'rewrite' => array( 'slug' => 'coach-method' ) ); register_taxonomy( 'coach-method', array( 'coach_mentor', 'post' ), $args ); // Coaching Technique $coach_technique = array( 'name' => _x( 'Coach Technique', 'taxonomy general name' ), 'singular_name' => _x( 'Coach Technique', 'taxonomy singular name' ), 'search_items' => __( 'Search in Coach Techniques' ), 'all_items' => __( 'All Coach Techniques' ), 'most_used_items' => null, 'parent_item' => null, 'parent_item_colon' => null, 'edit_item' => __( 'Edit Coach Technique' ), 'update_item' => __( 'Update Coach Technique' ), 'add_new_item' => __( 'Add new Coach Technique' ), 'new_item_name' => __( 'New Coach Technique' ), 'menu_name' => __( 'Coach Technique' ), ); $args = array( 'hierarchical' => false, 'labels' => $coach_technique, 'show_ui' => true, 'query_var' => true, 'rewrite' => array( 'slug' => 'coach-technique' ) ); register_taxonomy('coach-technique', array( 'coach_mentor' ), $args ); } add_action( 'init', 'themename_custom_taxonomies', 0 );
The second taxonomy is registering ‘coach-technique’ and is only being assigned to one custom post type, it’s hierarchical value is set to false so it will appear as tags – other than that the format is the same.
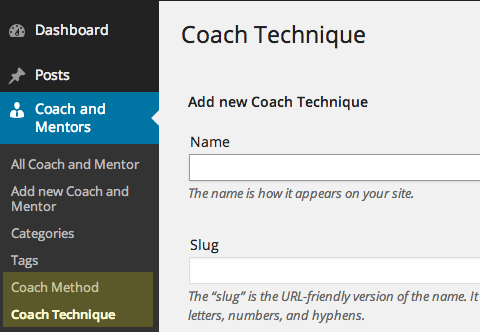
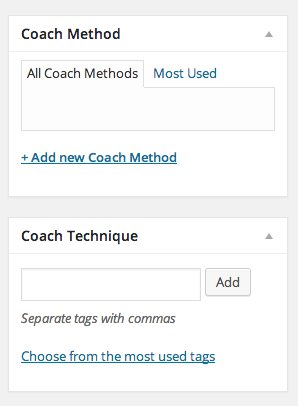
Flushing the Permalinks
Once you have set up the Taxonomies you will need to flush the permalinks cache by paying a visit to WP Admin > Settings > Permalinks as otherwise the new Taxonomy urls may not appear.
Custom Taxonomy Templates
To create custom templates for the taxonomy, you can target all taxonomy templates by using taxonomy.php or to get more granular you can target each taxonomy term by naming the templates taxonomy-‘taxonomyterm’.php, just swap out taxonomyterm with the actual term. So in the above example the 2 taxonomies can be targetted with taxonomy-coach-method.php and taxonomy-technique-method.php
Displaying the Custom Taxonomy in WordPress Templates
In the front end templates – this is what we would need to add to your loop to display the custom taxonomy near you existing post meta information.
<?php echo get_the_term_list( $post->ID, 'people', 'People: ', ', ', '' ); ?>
This uses the get_the_term_list function followed by 5 parameters, the post id which is required, the name of the taxonomy, a before label, a separator and an after label.
<?php get_the_term_list( $id, $taxonomy, $before, $sep, $after ) ?>
Here is a full gist example that uses both ingredients and recipes as tags and categories, you can just change or remove what you don’t need.