jQuery replace an image on click
jQuery replace an image on click- example below, this is a method to swap numerous images in HTML by manipulating the images src attribute value from the click of another element such as a button.
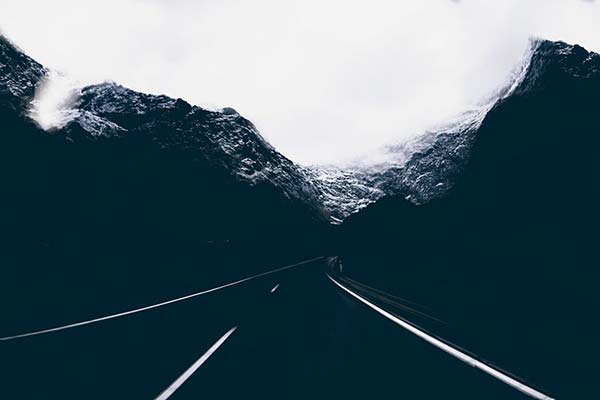
HTML
<div class="button-container"> <button class="black-button"></button> <button class="red-button"></button> <button class="blue-button"></button> <button class="yellow-button"></button> </div> <img id="change-image" src="/wp-content/uploads/2018/09/black.jpg" alt="Black Image" />
jQuery
jQuery(document).ready(function($){ $('.black-button').on({ 'click': function(){ $('#change-image').attr('src','/wp-content/uploads/2018/09/black.jpg'); } }); $('.red-button').on({ 'click': function(){ $('#change-image').attr('src','/wp-content/uploads/2018/09/red.jpg'); } }); $('.blue-button').on({ 'click': function(){ $('#change-image').attr('src','/wp-content/uploads/2018/09/blue.jpg'); } }); $('.yellow-button').on({ 'click': function(){ $('#change-image').attr('src','/wp-content/uploads/2018/09/yellow.jpg'); } }); });
Each button’s CSS class is targetted on click which then targets the ID of the image that changes the src attribute of the image.
This uses jQuerys attr() method which can change the value of a given attribute – so also here the alt text value can be changed for each image.
6 comments
Tony Pollard
yo this does not work big dawg
Danilo Battaglia
Hello,
is there a plugin which will do what has been done with coding?
Thank you
Dan
yourblogcoach
how can we swap images of gallery in random order?
Javier G
Hi, could it be possible to add a link to the image that gets displayed after clicking the button?
Sajeer.
Hi..m new in html..
I know to add “button”in html page..but i dont know to make the button select images from my gallery….so please can u teach a bit…
Thank u…
Jacques
Sajeer
i know it a bit late but you need to install/run jquery on your page.
put this
above your which contains the jquery then it will work